2、我方移动(man)
这需要把man从库中加载到mc中,然后设定它的位置,(注意:给定的位置只能在路上)
function attachMan(x, y) {
boxes.attachMovie('man', 'man', 800);
boxes.man._x = 10+(x*20);
boxes.man._y = 10+(y*20);
boxes.man.health = 100;
boxes.man.money = 0;
boxes.man.onEnterFrame = function() {
this.gotoAndStop(_root.mov);
};
}
这里面的x,y是给定的二维数组的下标。man还有自定义的两个属性:health,money;很明显一个是健康度一个是金币数。
man的移动是通过注册的监听对象来监听键盘事件来控制的。
键盘的一些ASCii对应的值:
90:"Z"
37:"left"
38:"up"
39:"right"
40:"down"
这里用到了一些摇摆因子来控制man的移动:
shootup:在键盘按下的情况下:只能发射三颗子弹,并且不能连发。
lpressed,upressed,dpressed,rpressed:控制man的移动。
在触发了按键后:数组motion里面被添加了新的数组值,这些值被按键的弹起事件所重新遍历,找到和按键对应的值,清空motion,最后执行m
oveOn函数来移动man。这样写固然复杂了一些,同样的功能比较简单的代码也能实现。
function movement() {
motion = new Array();
mcl = new Object();
mcl.onKeyDown = function() {
if (Key.getCode() == 90) {
if (boxes.man.health>0) {//如果生命〉0
if (shootup) {//摇摆因子
shootup = false;
if (bullet<3) {//一次只能发射三枚子弹
shoot();//发射函数。
}
}
}
}
if (Key.getCode() == 37) {
if (!lpressed) {//摇摆因子
lpressed = true;
l = motion.length;//
motion[l] = ['x', -1, -8, 0];
}
} else if (Key.getCode() == 38) {
if (!upressed) {
upressed = true;
u = motion.length;
motion[u] = ['y', -1, 0, -8];
}
} else if (Key.getCode() == 39) {
if (!rpressed) {
rpressed = true;
r = motion.length;
motion[r] = ['x', 1, 8, 0];
}
} else if (Key.getCode() == 40) {
if (!dpressed) {
dpressed = true;
d = motion.length;
motion[d] = ['y', 1, 0, 8];
}
}
};
mcl.onKeyUp = function() {
if (Key.getCode() == 90) {
shootup = true;
}
if (Key.getCode() == 37) {
for (z=0; z<motion.length; z++) {//遍历数组
if (motion[z][0] == 'x' and motion[z][1] == -1) {//得到相应的按键所存储的值
dirx = motion[z][2];//保存该值
diry = motion[z][3];
motion.splice(z, 1);//清除motion
lpressed = false;//停止移动;
}
}
} else if (Key.getCode() == 38) {
for (z=0; z<motion.length; z++) {
if (motion[z][0] == 'y' and motion[z][1] == -1) {
dirx = motion[z][2];
diry = motion[z][3];
motion.splice(z, 1);
upressed = false;
}
}
} else if (Key.getCode() == 39) {
for (z=0; z<motion.length; z++) {
if (motion[z][0] == 'x' and motion[z][1] == 1) {
dirx = motion[z][2];
diry = motion[z][3];
motion.splice(z, 1);
rpressed = false;
}
}
} else if (Key.getCode() == 40) {
for (z=0; z<motion.length; z++) {
if (motion[z][0] == 'y' and motion[z][1] == 1) {
dirx = motion[z][2];
diry = motion[z][3];
motion.splice(z, 1);
dpressed = false;
}
}
}
};
Key.addListener(mcl);
onEnterFrame = function () { moveOn(motion[0][0], motion[0][1]);};
}
//man的移动当然不是随意的,它有很多的限制和判断再里面。
在这里面有一个位置和数组下标的换算,换算成数组的下标以后才能根据数组中的值来判断他所在的“真实地图中的地形”。
function moveOn(a, b) {
if (a == 'x' and b == 1) {//如果右键被按下
for (l=-1; l<3; l += 2) {//循环的作用是判断它的上下两个位置是否是墙壁。
tempp = boxes.man._x+(boxes.man._width/2)+speed;//
tempo = (tempp-(boxes.man._width))/(boxes.man._width*2);//换算成数组的下标j
templ = boxes.man._y+((boxes.man._height/2)*l);
tempk = (templ-(boxes.man._height))/(boxes.man._height*2);////换算成数组的下标i
if (boxes['b'+Math.round(tempk)+'_'+Math.round(tempo)]._currentframe == 2 or
boxes['b'+Math.round(tempk)+'_'+Math.round(tempo)]._currentframe == 5) {
right++;
}
//根据当前的box所在的帧数来判断。
}
if (right != 0) {//如果前面不是道路
} else {
boxes.man._x += speed*b;//移动
left = 0;
up = 0;
down = 0;
mov = 1;
//修改其他方向的变量为0;
}
} else if (a == 'x' and b == -1) {
for (l=-1; l<3; l += 2) {
tempp = boxes.man._x-(boxes.man._width/2)-speed;
tempo = (tempp-(boxes.man._width))/(boxes.man._width*2);
templ = boxes.man._y+((boxes.man._height/2)*l);
tempk = (templ-(boxes.man._height))/(boxes.man._height*2);
if (boxes['b'+Math.round(tempk)+'_'+Math.round(tempo)]._currentframe == 2 or
boxes['b'+Math.round(tempk)+'_'+Math.round(tempo)]._currentframe == 5) {
left++;
}
}
if (left != 0) {
} else {
boxes.man._x += speed*b;
right = 0;
up = 0;
down = 0;
mov = 3;
}
} else if (a == 'y' and b == 1) {
for (l=-1; l<3; l += 2) {
tempp = boxes.man._x+((boxes.man._width/2)*l);
tempo = (tempp-(boxes.man._width))/(boxes.man._width*2);
templ = boxes.man._y+(boxes.man._height/2)+speed;
tempk = (templ-(boxes.man._height))/(boxes.man._height*2);
if (boxes['b'+Math.round(tempk)+'_'+Math.round(tempo)]._currentframe == 2 or
boxes['b'+Math.round(tempk)+'_'+Math.round(tempo)]._currentframe == 5) {
down++;
}
}
if (down != 0) {
} else {
boxes.man._y += speed*b;
left = 0;
up = 0;
right = 0;
mov = 2;
}
} else if (a == 'y' and b == -1) {
for (l=-1; l<3; l += 2) {
tempp = boxes.man._x+((boxes.man._width/2)*l);
tempo = (tempp-(boxes.man._width))/(boxes.man._width*2);
templ = boxes.man._y-(boxes.man._height/2)-speed;
tempk = (templ-(boxes.man._height))/(boxes.man._height*2);
if (boxes['b'+Math.round(tempk)+'_'+Math.round(tempo)]._currentframe == 2 or
boxes['b'+Math.round(tempk)+'_'+Math.round(tempo)]._currentframe == 5) {
up++;
}
}
if (up != 0) {
} else {
boxes.man._y += speed*b;
left = 0;
down = 0;
right = 0;
mov = 4;
}
}
}
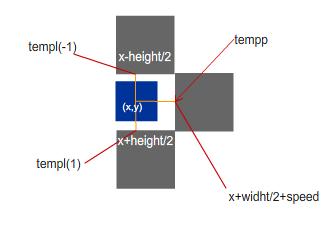
回复Comments
作者:
{commentrecontent}